|
||
---|---|---|
.. | ||
index.js | ||
license | ||
package.json | ||
readme.md |
readme.md
file-type 
Detect the file type of a Buffer/Uint8Array
The file type is detected by checking the magic number of the buffer.
Install
$ npm install file-type
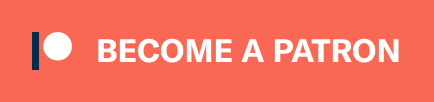
Usage
Node.js
const readChunk = require('read-chunk');
const fileType = require('file-type');
const buffer = readChunk.sync('unicorn.png', 0, fileType.minimumBytes);
fileType(buffer);
//=> {ext: 'png', mime: 'image/png'}
Or from a remote location:
const http = require('http');
const fileType = require('file-type');
const url = 'http://assets-cdn.github.com/images/spinners/octocat-spinner-32.gif';
http.get(url, response => {
response.on('readable', () => {
const chunk = response.read(fileType.minimumBytes);
response.destroy();
console.log(fileType(chunk));
//=> {ext: 'gif', mime: 'image/gif'}
});
});
Browser
const xhr = new XMLHttpRequest();
xhr.open('GET', 'unicorn.png');
xhr.responseType = 'arraybuffer';
xhr.onload = () => {
fileType(new Uint8Array(this.response));
//=> {ext: 'png', mime: 'image/png'}
};
xhr.send();
API
fileType(input)
Returns an Object
with:
ext
- One of the supported file typesmime
- The MIME type
Or null
when no match.
input
Type: Buffer
Uint8Array
It only needs the first .minimumBytes
bytes. The exception is detection of docx
, pptx
, and xlsx
which potentially requires reading the whole file.
fileType.minimumBytes
Type: number
The minimum amount of bytes needed to detect a file type. Currently, it's 4100 bytes, but it can change, so don't hardcode it.
Supported file types
jpg
png
gif
webp
flif
cr2
tif
bmp
jxr
psd
zip
tar
rar
gz
bz2
7z
dmg
mp4
m4v
mid
mkv
webm
mov
avi
wmv
mpg
mp2
mp3
m4a
ogg
opus
flac
wav
qcp
amr
pdf
epub
mobi
- Mobipocketexe
swf
rtf
woff
woff2
eot
ttf
otf
ico
flv
ps
xz
sqlite
nes
crx
xpi
cab
deb
ar
rpm
Z
lz
msi
mxf
mts
wasm
blend
bpg
docx
pptx
xlsx
3gp
jp2
- JPEG 2000jpm
- JPEG 2000jpx
- JPEG 2000mj2
- Motion JPEG 2000aif
odt
- OpenDocument for word processingods
- OpenDocument for spreadsheetsodp
- OpenDocument for presentationsxml
heic
cur
ktx
ape
- Monkey's Audiowv
- WavPackasf
- Advanced Systems Formatwma
- Windows Media Audiowmv
- Windows Media Videodcm
- DICOM Image Filempc
- Musepack (SV7 & SV8)ics
- iCalendarglb
- GL Transmission Format
SVG isn't included as it requires the whole file to be read, but you can get it here.
Pull request welcome for additional commonly used file types.
Related
- file-type-cli - CLI for this module
Created by
License
MIT